Introduction
Base64 is the most common encoding method for 8Bit byte on the internet. It is used to convert bytes into ASCII characters. It can also be used for simple encryption for an extra slim layer of protection (just by a little). Python can import Base64 as well and here is how to use Base64 in Python.
How does Base64 work:
Everytime when we use Base64 the following list is generated:
['A', 'B', 'C', ... 'a', 'b', 'c', ... '0', '1', ... '+', '/']
As the Base64 index table, it used "A-Z、a-z、0-9、+、/" 64 printable chars in total as the standard Base64 protocol. Each char represents 6 bits of data.
Steps to encode:
- Get the ASCII value of each character in the string
- Compute the 8-bit binary equivalent of the ASCII values
- Convert the 8-bit characters chunk into chunks of 6 bits by re-grouping the digits
- Convert the 6-bit binary groups to their respective decimal values
- Use the Base64 encoding table to align the respective Base64 values for each decimal value
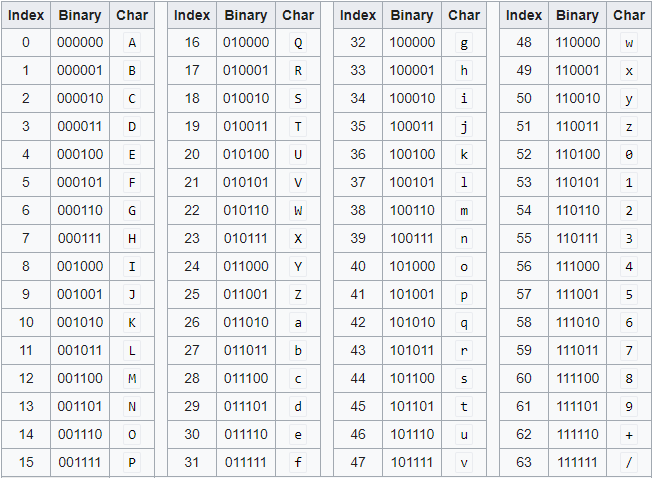
The Functions
Adding the functions and import:
# Adding the import
import base64
# Encoder and decoder functions of base64 providing a string
def encoding(info: str) -> str:
info_bytes = info.encode("ascii")
base64_bytes = base64.b64encode(info_bytes)
encrypted = base64_bytes.decode("ascii")
return encrypted
def decoding(info: str) -> str:
base64_bytes = info.encode("ascii")
info_bytes = base64.b64decode(base64_bytes)
decrypted = info_bytes.decode("ascii")
return decrypted
Usage
- Pass the string to encrypt to encoding function, store the returned encoded string.
- Pass the string to decrypt to decoding function, store the returned decoded string.
Example
- The following will translate based on base64 functions:
# Base64 encoded with encoding function UmljaGFyZCBMdQ== NDM3eHh4eHh4eA== QCE= Tm8gYmlydGhkYXkgYWRkZWQ=
# Base64 decoded with decoding function Richard Lu 437xxxxxxx @! No birthday added
Reference:
GeeksforGeeks
Comments 22 comments
KUBET được biết đến là một trong những cổng game hàng đầu và uy tín nhất tại Việt Nam, thu hút và nhận được sự tin tưởng của hàng triệu người chơi trên khắp cả nước. Nhà cái KUBET không chỉ đơn thuần nổi bật với sự phong phú và đa dạng trong các loại hình trò chơi giải trí mà còn gây ấn tượng mạnh mẽ bởi việc tạo ra một môi trường giải trí an toàn, đảm bảo chất lượng cao. Sự kết hợp giữa việc cung cấp nhiều lựa chọn trò chơi hấp dẫn và cam kết về tính bảo mật, an toàn đã giúp KU BET trở thành điểm đến lý tưởng cho những ai đang tìm kiếm một trải nghiệm giải trí toàn diện và đáng tin cậy. https://kubet-casino.org/
OKVIP là liên minh giải trí trực tuyến hàng đầu châu Á, cung cấp các trò chơi chất lượng cao với đồ họa đẹp và dịch vụ hỗ trợ 24/7. Hệ sinh thái đa dạng cùng cam kết bảo mật tuyệt đối, giúp OKVIP trở thành điểm đến giải trí uy tín cho người dùng. https://139.59.222.230/
The comment is private
VN88 da xuat hien va phat trien tren thi truong ca cuoc tu nam 2019 den nay, tich luy duoc gan 4 nam kinh nghiem hoat dong.
Tu ngay khi ra mat, VN88 da nhan duoc su danh gia cao va su ua chuong cua dong dao nguoi choi, chinh nho chat luong hang dau vao nam 2023.
Hay kham pha the gioi ca cuoc chuyen nghiep tai VN 88 ngay hom nay!
The comment is private
The comment is private
Chao mung ban den voi nha cai ca cuoc 8DAY – nen tang casino truc tuyen hang dau Viet Nam 2024. Kham pha ngay bay gio de co co hoi trai nghiem thien duong doi thuong hot nhat hien nay. Hang ngan uu dai khung dang cho dan choi den nhat.
The comment is private
If you’re a fan of fast-paced, high-energy driving games, Drift Boss is the perfect game to satisfy your need for speed. With the upgraded version now available at DriftBoss.org, the drifting experience has been taken to the next level, offering smoother gameplay and exciting new features. driftboss.org
Are you ready for the ultimate gaming challenge? Visit slope unblocked to experience the upgraded version of Slope Unblocked, a game that tests your reflexes and precision like never before. Website: https://slopeunblocked.biz/
Sao79 Club or Sao Win is a reputable card game portal with more than 500,000 downloads by 2024. Enjoy playing card games, Sic Bo, coin toss, fish shooting, lottery… Sao79 allows cross-platform transactions via Momo Pay, scanned bank codes, transfer, Zalo Pay, scratch cards… along with quick withdrawals to bank accounts, ATM cards. https://sao79.vip/
69VN là nhà cái cá cược trực tuyến hàng đầu tại Việt Nam, mang đến trải nghiệm giải trí đẳng cấp với hơn 500 trò chơi đa dạng như cá cược thể thao, casino trực tuyến, bắn cá, nổ hũ và game bài. 69vnpro.net
If you’re searching for a thrilling and engaging online gaming experience, look no further than Dino Game. This exciting platform offers a wide variety of games designed to entertain and challenge players of all ages and skill levels.
Welcome to cooking camp! 🌿🔥 Here, I share the joy of cooking in nature, embracing the peaceful rhythm of nature ASMR sounds. No rush, no noise – just the crackling fire, Camping ASMR the whisper of the wind and the pure flavor of outdoor life. Sit back, relax and enjoy the experience with me!
Are you looking for a fun and relaxing game to pass the time? Tiny Fishing is an ideal choice, offering simple yet engaging gameplay that lets you enjoy the thrill of fishing without any stress.
Welcome to Planet Clicker, the ultimate destination for fans of space exploration and idle gaming!
https://mpo188-login.sumbergading.id
https://mpo188-login.sumbergading.id
123B là nhà cái uy tín với cá cược thể thao, game bài, bắn cá, slot game hấp dẫn. Giao diện thân thiện, bảo mật cao, tỷ lệ kèo tốt, hỗ trợ 24/7. Trải nghiệm ngay tại 123Bsam.co.uk!
Göz yorgunluğunu azaltmak veya Zoom görüşmeleri için ışık testi yapmak istiyorsanız, Blackscreen.space harika bir araçtır. Ayrıca siyah ekran görselini indirebilirsiniz.
789bet là nhà cái uy tín, được cấp phép bởi PAGCOR, thu hút hơn 10 triệu thành viên. Đăng ký ngay để nhận quà tặng hấp dẫn và trải nghiệm cá cược an toàn, chất lượng.
789bet là nhà cái uy tín, được cấp phép bởi PAGCOR, thu hút hơn 10 triệu thành viên. Đăng ký ngay để nhận quà tặng hấp dẫn và trải nghiệm cá cược an toàn, chất lượng.